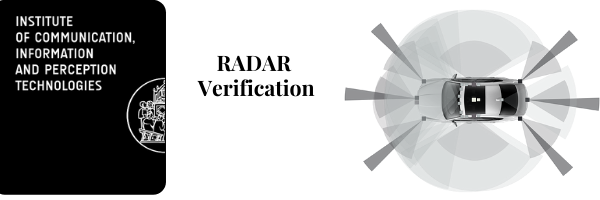 |
TI-radar AWR1843 C674x DSP core
1
|
Go to the documentation of this file.
39 #ifndef DSS_CONFIG_EDMA_UTIL_H
40 #define DSS_CONFIG_EDMA_UTIL_H
42 #include <ti/drivers/edma/edma.h>
101 bool isEventTriggered,
102 uint16_t shadowParamId,
107 uint8_t eventQueueId,
108 EDMA_transferCompletionCallbackFxn_t transferCompletionCallbackFxn,
109 uintptr_t transferCompletionCallbackFxnArg);
162 bool isEventTriggered,
163 uint16_t shadowParamId,
164 uint16_t sampleLenInBytes,
165 uint16_t numRangeBins,
168 uint16_t numDopplerBins,
169 uint8_t eventQueueId,
170 EDMA_transferCompletionCallbackFxn_t transferCompletionCallbackFxn,
171 uintptr_t transferCompletionCallbackFxnArg);
209 bool isEventTriggered,
210 uint16_t shadowParamId,
215 uint8_t eventQueueId,
216 EDMA_transferCompletionCallbackFxn_t transferCompletionCallbackFxn,
217 uintptr_t transferCompletionCallbackFxnArg);
239 uint8_t triggerEnabled);
int32_t EDMAutil_configType1(EDMA_Handle handle, uint8_t *srcBuff, uint8_t *dstBuff, uint8_t chId, bool isEventTriggered, uint16_t shadowParamId, uint16_t aCount, uint16_t bCount, int16_t srcBIdx, int16_t dstBIdx, uint8_t eventQueueId, EDMA_transferCompletionCallbackFxn_t transferCompletionCallbackFxn, uintptr_t transferCompletionCallbackFxnArg)
int32_t EDMAutil_configType2a(EDMA_Handle handle, uint8_t *srcBuff, uint8_t *dstBuff, uint8_t chId, bool isEventTriggered, uint16_t shadowParamId, uint16_t sampleLenInBytes, uint16_t numRangeBins, uint8_t numTxAnt, uint8_t numRxAnt, uint16_t numDopplerBins, uint8_t eventQueueId, EDMA_transferCompletionCallbackFxn_t transferCompletionCallbackFxn, uintptr_t transferCompletionCallbackFxnArg)
int32_t EDMAutil_triggerType3(EDMA_Handle handle, uint8_t *srcBuff, uint8_t *dstBuff, uint8_t chId, uint8_t triggerEnabled)
int32_t EDMAutil_configType3(EDMA_Handle handle, uint8_t *srcBuff, uint8_t *dstBuff, uint8_t chId, bool isEventTriggered, uint16_t shadowParamId, uint16_t aCount, uint16_t bCount, int16_t srcBIdx, int16_t destBIdx, uint8_t eventQueueId, EDMA_transferCompletionCallbackFxn_t transferCompletionCallbackFxn, uintptr_t transferCompletionCallbackFxnArg)