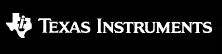 |
 |
53 #define RL_MAX_SB_IN_MSG (32U) 54 #define RL_GET_UNIQUE_SBID(x,y) (((x)* RL_MAX_SB_IN_MSG) + (y)) 55 #define RL_GET_SBID_FROM_MSG(x,y) ((x) - (RL_MAX_SB_IN_MSG *(y))) 56 #define RL_GET_MSGID_FROM_SBID(x) ((x) / RL_MAX_SB_IN_MSG) 57 #define RL_GET_SBID_FROM_UNIQ_SBID(x) ((x) % RL_MAX_SB_IN_MSG) 59 #define RL_SBC_ID_INDEX (0U) 60 #define RL_SBC_ID_SIZE (2U) 61 #define RL_SBC_LEN_INDEX (RL_SBC_ID_INDEX + RL_SBC_ID_SIZE) 62 #define RL_SBC_LEN_SIZE (2U) 63 #define RL_SBC_PL_INDEX (RL_SBC_LEN_INDEX + RL_SBC_LEN_SIZE) 64 #define RL_MIN_SBC_LEN (RL_SBC_LEN_SIZE + RL_SBC_ID_SIZE) 75 #define RL_RF_RESP_ERROR_MSG (0x00U) 76 #define RL_RF_RESERVED0_MSG (0x01U) 77 #define RL_RF_RESERVED1_MSG (0x02U) 78 #define RL_RF_RESERVED2_MSG (0x03U) 79 #define RL_RF_STATIC_CONF_SET_MSG (0x04U) 80 #define RL_RF_STATIC_CONF_GET_MSG (0x05U) 81 #define RL_RF_INIT_MSG (0x06U) 82 #define RL_RF_RESERVED3_MSG (0x07U) 83 #define RL_RF_DYNAMIC_CONF_SET_MSG (0x08U) 84 #define RL_RF_DYNAMIC_CONF_GET_MSG (0x09U) 85 #define RL_RF_FRAME_TRIG_MSG (0x0AU) 86 #define RL_RF_RESERVED4_MSG (0x0BU) 87 #define RL_RF_ADVANCED_FEATURES_SET_MSG (0x0CU) 88 #define RL_RF_ADVANCED_FEATURES_GET_MSG (0x0DU) 89 #define RL_RF_MONITORING_CONF_SET_MSG (0x0EU) 90 #define RL_RF_MONITORING_CONF_GET_MSG (0x0FU) 91 #define RL_RF_RESERVED5_MSG (0x10U) 92 #define RL_RF_STATUS_GET_MSG (0x11U) 93 #define RL_RF_RESERVED6_MSG (0x12U) 94 #define RL_RF_MONITORING_REPO_GET_MSG (0x13U) 95 #define RL_RF_RESERVED7_MSG (0x14U) 96 #define RL_RF_RESERVED8_MSG (0x15U) 97 #define RL_RF_MISC_CONF_SET_MSG (0x16U) 98 #define RL_RF_MISC_CONF_GET_MSG (0x17U) 99 #define RL_RF_ASYNC_EVENT_MSG (0x80U) 100 #define RL_RF_ASYNC_EVENT_1_MSG (0x81U) 104 #define RL_DSP_RESERVED0_MSG (0x100U) 105 #define RL_DSP_RESERVED1_MSG (0x101U) 106 #define RL_DSP_CONFIG_SET_MSG (0x102U) 107 #define RL_DSP_CONFIG_GET_MSG (0x103U) 108 #define RL_DSP_RESERVED2_MSG (0x104U) 109 #define RL_DSP_RESERVED3_MSG (0x105U) 110 #define RL_DSP_CONFIG_APPLY_MSG (0x106U) 111 #define RL_DSP_STATUS_GET_MSG (0x107U) 113 #define RL_DSP_ASYNC_EVENT_MSG (0x180U) 116 #define RL_DEV_POWERUP_MSG (0x200U) 117 #define RL_DEV_RESERVED1_MSG (0x201U) 118 #define RL_DEV_CONFIG_SET_MSG (0x202U) 119 #define RL_DEV_CONFIG_GET_MSG (0x203U) 120 #define RL_DEV_FILE_DOWNLOAD_MSG (0x204U) 121 #define RL_DEV_RESERVED2_MSG (0x205U) 122 #define RL_DEV_CONFIG_APPLY_MSG (0x206U) 123 #define RL_DEV_STATUS_GET_MSG (0x207U) 124 #define RL_DEV_MONITORING_CONF_SET_MSG (0x208U) 125 #define RL_DEV_MONITORING_CONF_GET_MSG (0x209U) 126 #define RL_DEV_RESERVED3_MSG (0x20AU) 127 #define RL_DEV_MONITORING_REPORT_GET_MSG (0x20BU) 128 #define RL_DEV_INTERNAL_CONF_SET_MSG (0x20CU) 129 #define RL_DEV_INTERNAL_CONF_GET_MSG (0x20DU) 130 #define RL_DEV_ASYNC_EVENT_MSG (0x280U) 137 #define RL_RF_RESP_ERROR_SB (0x0U) 138 #define RL_RF_RESP_MAX (0x1U) 144 #define RL_RF_CHAN_CONF_SB (0x0U) 145 #define RL_RF_RESERVED_SB (0x1U) 146 #define RL_RF_ADCOUT_CONF_SB (0x2U) 147 #define RL_RF_LOWPOWERMODE_CONF_SB (0x3U) 148 #define RL_RF_DYNAMICPOWERSAVE_CONF_SB (0x4U) 149 #define RL_RF_HIGHSPEEDINTFCLK_CONF_SET_SB (0x5U) 150 #define RL_RF_DEVICE_CFG_SB (0x6U) 151 #define RL_RF_RADAR_MISC_CTL_SB (0x7U) 152 #define RL_RF_CAL_MON_FREQ_LIMIT_SB (0x8U) 153 #define RL_RF_INIT_CALIB_CONF_SB (0x9U) 154 #define RL_RF_TX_FREQ_PWR_LIMIT_SB (0xAU) 155 #define RL_RF_CAL_DATA_RD_WR_SB (0xBU) 156 #define RL_RF_PH_SHIFT_CAL_DATA_RD_WR_SB (0xCU) 157 #define RL_RF_STAT_CONF_SB_MAX (0xDU) 164 #define RL_RF_RF_INIT_SB (0x0U) 165 #define RL_RF_INIT_SB_MAX (0x1U) 171 #define RL_RF_PROFILE_CONF_SB (0x0U) 172 #define RL_RF_CHIRP_CONF_SB (0x1U) 173 #define RL_RF_FRAME_CONF_SB (0x2U) 174 #define RL_RF_CONT_STREAMING_MODE_CONF_SB (0x3U) 175 #define RL_RF_CONT_STREAMING_MODE_EN_SB (0x4U) 176 #define RL_RF_FRAME_CONF_ADVANCED_SB (0x5U) 177 #define RL_RF_PERCHIRPPHASESHIFT_CONF_SB (0x6U) 178 #define RL_RF_PROG_FILT_COEFF_RAM_SET_SB (0x7U) 179 #define RL_RF_PROG_FILT_CONF_SET_SB (0x8U) 180 #define RL_RF_CALIB_MON_TIME_UNIT_SB (0x9U) 181 #define RL_RF_RUN_TIME_CALIB_CONF_TRIG_SB (0xAU) 182 #define RL_RF_INTER_RX_GAIN_PH_FREQ_CTRL_SB (0xBU) 183 #define RL_RF_RX_GAIN_TEMPLUT_CONF_SB (0xCU) 184 #define RL_RF_TX_GAIN_TEMPLUT_CONF_SB (0xDU) 185 #define RL_RF_LB_BURST_CFG_SET_SB (0xEU) 186 #define RL_RF_DYN_CHIRP_CFG_SET_SB (0xFU) 187 #define RL_RF_DYN_PERCHIRP_PHSHFT_CFG_SET_SB (0x10U) 188 #define RL_RF_DYN_CHIRP_CFG_EN_SB (0x11U) 189 #define RL_RF_INTERCHIRP_BLOCK_CTRL_SB (0x12U) 190 #define RL_RF_SUBFRAME_START_SB (0x13U) 191 #define RL_RF_RESERVED0 (0x14U) 192 #define RL_RF_DYNAMIC_CONF_SB_MAX (0x15U) 198 #define RL_RF_FRAMESTARTSTOP_CONF_SB (0x0U) 199 #define RL_RF_FRAME_TRIG_SB_MAX (0x1U) 205 #define RL_RF_BPM_COMMON_CONF_SB (0x0U) 206 #define RL_RF_BPM_CHIRP_CONF_SB (0x1U) 207 #define RL_RF_BPM_LFSR_CONF_SB (0x2U) 208 #define RL_RF_BPM_PROGSEQ_CONF_SB (0x3U) 209 #define RL_RF_INTERFERENCEDET_CONF_SB (0x4U) 210 #define RL_RF_BUMPEREST_CONF_SB (0x5U) 211 #define RL_RF_ADV_FEATURES_SB_MAX (0x6U) 217 #define RL_RF_MONITORING_CONF_SB (0x0U) 218 #define RL_RF_MONIT_CONF_SB_MAX (0x1U) 224 #define RL_RF_RFVERSION_SB (0x0U) 225 #define RL_RF_RFCPUFAULT_STATUS_SB (0x1U) 226 #define RL_RF_RFESMFAULT_STATUS_SB (0x2U) 227 #define RL_RF_DIEID_STATUS_SB (0x3U) 228 #define RL_RF_BOOTUP_BIST_STATUS_SB (0x4U) 229 #define RL_RF_STATUS_SB_MAX (0x5U) 235 #define RL_RF_MONITORING_REPO_GET_SB (0x0U) 236 #define RL_RF_MONITORING_REPO_GET_SB_MAX (0x1U) 242 #define RL_RF_DIG_MON_EN_SB (0x0U) 243 #define RL_RF_DIG_MON_PERIODIC_CONF_SB (0x1U) 244 #define RL_RF_ANA_MON_EN_SB (0x2U) 245 #define RL_RF_TEMP_MON_CONF_SB (0x3U) 246 #define RL_RF_RX_GAIN_PHASE_MON_CONF_SB (0x4U) 247 #define RL_RF_RX_NOISE_MON_CONF_SB (0x5U) 248 #define RL_RF_RX_IFSTAGE_MON_CONF_SB (0x6U) 249 #define RL_RF_TX0_POW_MON_CONF_SB (0x7U) 250 #define RL_RF_TX1_POW_MON_CONF_SB (0x8U) 251 #define RL_RF_TX2_POW_MON_CONF_SB (0x9U) 252 #define RL_RF_TX0_BALLBREAK_MON_CONF_SB (0xAU) 253 #define RL_RF_TX1_BALLBREAK_MON_CONF_SB (0xBU) 254 #define RL_RF_TX2_BALLBREAK_MON_CONF_SB (0xCU) 255 #define RL_RF_TX_GAIN_PHASE_MISMATCH_MON_CONF_SB (0xDU) 256 #define RL_RF_TX0_BPM_MON_CONF_SB (0xEU) 257 #define RL_RF_TX1_BPM_MON_CONF_SB (0xFU) 258 #define RL_RF_TX2_BPM_MON_CONF_SB (0x10U) 259 #define RL_RF_SYNTH_FREQ_MON_CONF_SB (0x11U) 260 #define RL_RF_EXT_ANA_SIGNALS_MON_CONF_SB (0x12U) 261 #define RL_RF_TX0_INT_ANA_SIGNALS_MON_CONF_SB (0x13U) 262 #define RL_RF_TX1_INT_ANA_SIGNALS_MON_CONF_SB (0x14U) 263 #define RL_RF_TX2_INT_ANA_SIGNALS_MON_CONF_SB (0x15U) 264 #define RL_RF_RX_INT_ANA_SIGNALS_MON_CONF_SB (0x16U) 265 #define RL_RF_PMCLKLO_INT_ANA_SIGNALS_MON_CONF_SB (0x17U) 266 #define RL_RF_GPADC_INT_ANA_SIGNALS_MON_CONF_SB (0x18U) 267 #define RL_RF_PLL_CONTROL_VOLT_MON_CONF_SB (0x19U) 268 #define RL_RF_DUAL_CLOCK_COMP_MON_CONF_SB (0x1AU) 269 #define RL_RF_RX_SATURATION_MON_CONF_SB (0x1BU) 270 #define RL_RF_RX_SIGIMG_MON_CONF_SB (0x1CU) 271 #define RL_RF_RX_MIXER_IN_POWR_CONF_SB (0x1DU) 272 #define RL_RF_SYNTH_LIN_MON_CONF_SB (0x1EU) 273 #define RL_RF_FAULT_INJECTION_CONF_SB (0x1FU) 279 #define RL_RF_ORBIT_ENABLE_SB (0x00U) 280 #define RL_RF_ORBIT_TEST_API_SB (0x01U) 281 #define RL_RF_TEST_SOURCE_CONFIG_SET_SB (0x02U) 282 #define RL_RF_TEST_SOURCE_ENABLE_SET_SB (0x03U) 283 #define RL_RF_DEBUG_API_SB (0x04U) 284 #define RL_RF_REG_CONFIG_SET_SB (0x05U) 285 #define RL_RF_TRIGGER_CALIB_MON_SB (0x06U) 286 #define RL_RF_CALIB_DISABLE_SB (0x07U) 287 #define RL_RF_MISC_CONF_SET_RESERVED_01 (0x08U) 288 #define RL_RF_MISC_CONF_SET_RESERVED_02 (0x09U) 289 #define RL_RF_CHAR_CONF_DYNAMIC_SET_SB (0x0AU) 290 #define RL_RF_TEMP_SENS_TRIM_SET_SB (0x0BU) 291 #define RL_RF_LDOBYPASS_SET_SB (0x0CU) 292 #define RL_RF_PALOOPBACK_CFG_SET_SB (0x0DU) 293 #define RL_RF_PSLOOPBACK_CFG_SET_SB (0x0EU) 294 #define RL_RF_IFLOOPBACK_CFG_SET_SB (0x0FU) 295 #define RL_RF_GPADC_CONF_SET_SB (0x10U) 296 #define RL_RF_PD_TRIM_1GHZ_SET_SB (0x11U) 297 #define RL_RF_MEAS_TX_POWER_SET_SB (0x12U) 298 #define RL_RF_MEAS_PD_POWER_SET_SB (0x13U) 299 #define RL_RF_MISC_CONF_SET_SB_MAX (0x14U) 305 #define RL_RF_MISC_CONF_GET_RESERVED1 (0x00U) 306 #define RL_RF_MISC_CONF_GET_RESERVED2 (0x01U) 307 #define RL_RF_TEST_SOURCE_CONFIG_GET_SB (0x02U) 308 #define RL_RF_TEST_SOURCE_ENABLE_GET_SB (0x03U) 309 #define RL_RF_MISC_CONF_GET_RESERVED3 (0x04U) 310 #define RL_RF_REG_CONFIG_GET_SB (0x05U) 311 #define RL_RF_MISC_CONF_GET_RESERVED4 (0x06U) 312 #define RL_RF_MISC_CONF_GET_RESERVED5 (0x07U) 313 #define RL_RF_MISC_CONF_GET_RESERVED6 (0x08U) 314 #define RL_RF_CHAR_CONF_STATIC_GET_SB (0x09U) 315 #define RL_RF_CHAR_CONF_DYNAMIC_GET_SB (0x0AU) 316 #define RL_RF_MISC_CONF_GET_RESERVED7 (0x0BU) 317 #define RL_RF_MISC_CONF_GET_SB_MAX (0x0CU) 324 #define RL_RF_AE_RESERVED1 (0x00U) 325 #define RL_RF_AE_RESERVED2 (0x01U) 326 #define RL_RF_AE_CPUFAULT_SB (0x02U) 327 #define RL_RF_AE_ESMFAULT_SB (0x03U) 328 #define RL_RF_AE_INITCALIBSTATUS_SB (0x04U) 329 #define RL_RF_AE_RESERVED3 (0x05U) 330 #define RL_RF_AE_RESERVED4 (0x06U) 331 #define RL_RF_AE_RESERVED5 (0x07U) 332 #define RL_RF_AE_RESERVED6 (0x08U) 333 #define RL_RF_AE_RESERVED7 (0x09U) 334 #define RL_RF_AE_RESERVED8 (0x0AU) 335 #define RL_RF_AE_FRAME_TRIGGER_RDY_SB (0x0BU) 336 #define RL_RF_AE_GPADC_MEAS_DATA_SB (0x0CU) 337 #define RL_RF_AE_RESERVED9 (0x0DU) 338 #define RL_RF_AE_RESERVED10 (0x0EU) 339 #define RL_RF_AE_FRAME_END_SB (0x0FU) 340 #define RL_RF_AE_ANALOG_FAULT_SB (0x10U) 341 #define RL_RF_AE_MON_TIMING_FAIL_REPORT_SB (0x11U) 342 #define RL_RF_AE_RUN_TIME_CALIB_REPORT_SB (0x12U) 343 #define RL_RF_AE_DIG_LATENTFAULT_REPORT_SB (0x13U) 344 #define RL_RF_AE_MON_DFE_STATISTICS_SB (0x14U) 345 #define RL_RF_AE_MON_REPORT_HEADER_SB (0x15U) 346 #define RL_RF_AE_MON_DIG_PERIODIC_REPORT_SB (0x16U) 347 #define RL_RF_AE_MON_TEMPERATURE_REPORT_SB (0x17U) 348 #define RL_RF_AE_MON_RX_GAIN_PHASE_REPORT (0X18U) 349 #define RL_RF_AE_MON_RX_NOISE_FIG_REPORT (0X19U) 350 #define RL_RF_AE_MON_RX_IF_STAGE_REPORT (0X1AU) 351 #define RL_RF_AE_MON_TX0_POWER_REPORT (0X1BU) 352 #define RL_RF_AE_MON_TX1_POWER_REPORT (0X1CU) 353 #define RL_RF_AE_MON_TX2_POWER_REPORT (0X1DU) 354 #define RL_RF_AE_MON_TX0_BALLBREAK_REPORT (0X1EU) 355 #define RL_RF_AE_MON_TX1_BALLBREAK_REPORT (0X1FU) 361 #define RL_RF_AE_MON_TX2_BALLBREAK_REPORT (0X00U) 362 #define RL_RF_AE_MON_TX_GAIN_MISMATCH_REPORT (0X01U) 363 #define RL_RF_AE_MON_TX0_BPM_REPORT (0X02U) 364 #define RL_RF_AE_MON_TX1_BPM_REPORT (0X03U) 365 #define RL_RF_AE_MON_TX2_BPM_REPORT (0X04U) 366 #define RL_RF_AE_MON_SYNTHESIZER_FREQ_REPORT (0X05U) 367 #define RL_RF_AE_MON_EXT_ANALOG_SIG_REPORT (0X06U) 368 #define RL_RF_AE_MON_TX0_INT_ANA_SIG_REPORT (0X07U) 369 #define RL_RF_AE_MON_TX1_INT_ANA_SIG_REPORT (0X08U) 370 #define RL_RF_AE_MON_TX2_INT_ANA_SIG_REPORT (0X09U) 371 #define RL_RF_AE_MON_RX_INT_ANALOG_SIG_REPORT (0X0AU) 372 #define RL_RF_AE_MON_PMCLKLO_INT_ANA_SIG_REPORT (0X0BU) 373 #define RL_RF_AE_MON_GPADC_INT_ANA_SIG_REPORT (0X0CU) 374 #define RL_RF_AE_MON_PLL_CONTROL_VOLT_REPORT (0X0DU) 375 #define RL_RF_AE_MON_DCC_CLK_FREQ_REPORT (0X0EU) 376 #define RL_RF_AE_MON_RESERVED0 (0x0FU) 377 #define RL_RF_AE_MON_RESERVED1 (0x10U) 378 #define RL_RF_AE_MON_RX_MIXER_IN_PWR_REPORT (0x11U) 379 #define RL_RF_AE_SB_MAX (0x12U) 386 #define RL_SYS_RF_POWERUP_SB (0x0U) 387 #define RL_SYS_NUM_OF_RF_POWERUP_SBC (0x1U) 393 #define RL_SYS_FILE_DWLD_SB (0x0U) 394 #define RL_SYS_NUM_OF_FILE_DWLD_SBC (0x1U) 400 #define RL_DEV_MCUCLOCK_CONF_SET_SB (0x00U) 401 #define RL_DEV_RX_DATA_FORMAT_CONF_SET_SB (0x01U) 402 #define RL_DEV_RX_DATA_PATH_CONF_SET_SB (0x02U) 403 #define RL_DEV_DATA_PATH_LANEEN_SET_SB (0x03U) 404 #define RL_DEV_DATA_PATH_CLOCK_SET_SB (0x04U) 405 #define RL_DEV_DATA_PATH_CFG_SET_SB (0x05U) 406 #define RL_DEV_RX_CONTSTREAMING_MODE_CONF_SET_SB (0x06U) 407 #define RL_DEV_CSI2_CFG_SET_SB (0x07U) 408 #define RL_DEV_PMICCLOCK_CONF_SET_SB (0x08U) 409 #define RL_DEV_PERIODICTESTS_CONF_SB (0x09U) 410 #define RL_DEV_LATENTFAULT_TEST_CONF_SB (0x0AU) 411 #define RL_DEV_TESTPATTERN_GEN_SET_SB (0x0BU) 412 #define RL_DEV_MISC_CFG_SET_SB (0x0CU) 413 #define RL_DEV_CONFIG_SB_MAX (0x0DU) 419 #define RL_SYS_CONFIG_APPLY_SB (0x0U) 420 #define RL_ADV_FRAME_DATA_CONFIG_SB (0x1U) 421 #define RL_SYS_CONFIG_APPLY_SB_MAX (0x2U) 427 #define RL_SYS_VERSION_SB (0x0U) 428 #define RL_SYS_CPUFAULT_STATUS_SB (0x1U) 429 #define RL_SYS_ESMFAULT_STATUS_SB (0x2U) 430 #define RL_SYS_FRAME_CONFIG_GET_SB (0x3U) 431 #define RL_SYS_ADV_FRAME_CONFIG_GET_SB (0x4U) 432 #define RL_SYS_NUM_OF_STATUS_SBC (0x5U) 438 #define RL_DEV_AE_MSSPOWERUPDONE_SB (0x0U) 439 #define RL_DEV_AE_RFPOWERUPDONE_SB (0x1U) 440 #define RL_DEV_AE_MSS_CPUFAULT_SB (0x2U) 441 #define RL_DEV_AE_MSS_ESMFAULT_SB (0x3U) 442 #define RL_DEV_AE_RESERVED0 (0x4U) 443 #define RL_DEV_AE_MSS_BOOTERRSTATUS_SB (0x5U) 444 #define RL_DEV_AE_MSS_LATENTFLT_TEST_REPORT_SB (0x6U) 445 #define RL_DEV_AE_MSS_PERIODIC_TEST_STATUS_SB (0x7U) 446 #define RL_DEV_AE_MSS_RF_ERROR_STATUS_SB (0x8U) 447 #define RL_DEV_AE_MSS_VMON_ERROR_STATUS_SB (0x9U) 448 #define RL_DEV_AE_MSS_ADC_DATA_SB (0xAU) 449 #define RL_DEV_AE_SB_MAX (0xBU) 454 #define RL_SYS_AE_DEV_CPUFAULT_SB (0x0U) 455 #define RL_SYS_AE_DEV_ESMFAULT_SB (0x1U) 456 #define RL_SYS_NUM_OF_AE_DEVFAULT_REPO_SBC (0x2U) 463 #define RL_RF_DFE_STATISTICS_REPORT_GET_SB (0x0U) 469 #define RL_DEV_MEM_REGISTER_SB (0x0U) 470 #define RL_DEV_NUM_OF_INTERN_CONF_SBC (0x1U) 476 #define RL_SYS_NRESP_INVLD_OPCODE (0x0001U) 477 #define RL_SYS_NRESP_INVLD_MSGID (0x0002U) 478 #define RL_SYS_NRESP_INVLD_NUM_SB (0x0003U) 479 #define RL_SYS_NRESP_INVLD_SB_ID (0x0004U) 480 #define RL_SYS_NRESP_INVLD_SB_LEN (0x0005U) 481 #define RL_SYS_NRESP_SB_GET_ERROR (0x0006U) 482 #define RL_SYS_NRESP_SB_SET_ERROR (0x0007U) 483 #define RL_SYS_NRESP_SB_SET_INVL_DATA (0x0008U) 484 #define RL_SYS_NRESP_CRC_FAILED (0x0009U) 485 #define RL_SYS_NRESP_FILETYPE_MISMATCHED (0x000AU) 487 #define RL_SYS_NRESP_REG_WRITE_FAILED (0x000BU) 488 #define RL_SYS_NRESP_ERROR_RESRVD (0xFFFFU)
Copyright 2018, Texas Instruments Incorporated