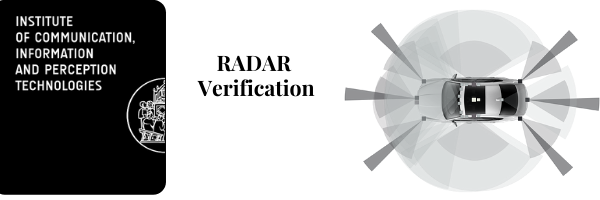 |
TI-radar AWR1843 C674x DSP core
1
|
Go to the documentation of this file.
39 #ifndef DSS_DATA_PATH_H
40 #define DSS_DATA_PATH_H
45 #include <ti/sysbios/knl/Semaphore.h>
48 #include <ti/common/sys_common.h>
49 #include <ti/common/mmwave_error.h>
50 #include <ti/drivers/adcbuf/ADCBuf.h>
51 #include <ti/drivers/edma/edma.h>
58 #define BYTES_PER_SAMP_1D (2*sizeof(int16_t))
59 #define BYTES_PER_SAMP_2D (2*sizeof(int32_t))
60 #define BYTES_PER_SAMP_DET sizeof(uint16_t)
63 #define DET_THRESH_MULT 25
64 #define DET_THRESH_SHIFT 5 //DET_THRESH_MULT and DET_THRESH_SHIFT together define the CFAR-CA threshold
65 #define DET_GUARD_LEN 4 // this is the one sided guard lenght
66 #define DET_NOISE_LEN 16 //this is the one sided noise length
68 #define PI_ 3.1415926535897
69 #define ONE_Q15 (1 << 15)
70 #define ONE_Q19 (1 << 19)
71 #define ONE_Q8 (1 << 8)
73 #define MMWDEMO_MEMORY_ALLOC_DOUBLE_WORD_ALIGN 8
74 #define MMWDEMO_MEMORY_ALLOC_MAX_STRUCT_ALIGN sizeof(uint64_t)
77 #define MMW_PEAK_GROUPING_DET_MATRIX_BASED 1
80 #define MMW_PEAK_GROUPING_CFAR_PEAK_BASED 2
83 #define MMW_NOISE_AVG_MODE_CFAR_CA ((uint8_t)0U)
86 #define MMW_NOISE_AVG_MODE_CFAR_CAGO ((uint8_t)1U)
89 #define MMW_NOISE_AVG_MODE_CFAR_CASO ((uint8_t)2U)
92 #define MMWDEMO_OUTPUT_MSG_CLUSTERS 2
93 #define MMWDEMO_OUTPUT_MSG_TRACKED_OBJECTS 3
94 #define MMWDEMO_OUTPUT_MSG_PARKING_ASSIST 4
214 uint16_t dopplerSNRdB;
294 #define DBSCAN_ERROR_CODE_OFFSET 100
376 #define TRACKER_SCRATCHPAD_FLT_SIZE (N_MEASUREMENTS + \
377 N_UNIQ_ELEM_IN_SYM_RESIDCOVMAT + \
378 N_UNIQ_ELEM_IN_SYM_RESIDCOVMAT + \
379 N_UNIQ_ELEM_IN_HMAT + \
380 (N_STATES*N_MEASUREMENTS) + \
381 N_UNIQ_ELEM_IN_SYM_COVMAT + \
382 (N_STATES*N_MEASUREMENTS) + \
383 (N_STATES*N_STATES) + \
384 N_UNIQ_ELEM_IN_SYM_COVMAT + \
386 #define TRACKER_SCRATCHPAD_SHORT_SIZE (MRR_MAX_OBJ_OUT + 2*MAX_TRK_OBJs)
907 float convertSNRdBToVar(uint16_t SNRdB,uint16_t bitW, uint16_t n_samples,
float resolution);
925 float convertSNRLinToVar(uint16_t SNRLin,uint16_t bitW, uint16_t n_samples,
float resolution);
1012 int32_t numDopplerBins,
1013 uint32_t *azimuthModCoefs,
1014 uint32_t *azimuthModCoefsThirdBin,
1015 uint32_t *azimuthModCoefsTwoThirdBin,
1041 float * azimuthMagSqr);
1065 float * azimuthMagSqr);
cmplx32ReIm_t * windowingBuf2D
window output for 2D FFT
uint16_t dopplerLineMaskLen
size of dopplerLineMask array, (number of 32_bit words, for example for Doppler FFT size of 64 this l...
cmplx16ImRe_t * rxChPhaseComp
Pointer to the Rx Gain phase compensation params.
int32_t MmwDemo_dataPathCopyEdmaHandle(DSS_DataPathObj *objOutput, DSS_DataPathObj *objInput)
These parameters allow the SNR requirements to be varied as a function of range.
EDMA_Handle edmaHandle[2]
Handle of the EDMA driver.
uint32_t interFrameCPULoad
CPU Load during inter frame period - i.e. after chirps are done and before next frame starts.
MmwDemo_objRaw2D_t * detObj2DRaw
Detected objects before peak grouping.
#define MAX_NUM_RANGE_DEPENDANT_SNR_THRESHOLDS
The number of SNR Thresholds - used to vary the SNR requirement as a function of range.
uint16_t dopplerSNRdB
Peak value.
uint16_t maxVelAssocThresh
uint16_t minPointsInCluster
cmplx32ReIm_t * fftOut2D
2D FFT output
int16_t * scratchPadShort
Structure for each cluster information report .
KFstate_t * trackerState
tracking state.
uint16_t rangelim
Range (in meters * (1 << xyzOutputQFormat)) upto which the SNR requirement is valid.
uint16_t maxRange
maximum range at which a target is detected ( in xyzOutputQFormat precision).
uint16_t peakVal
Peak value.
float convertSNRdBToVar(uint16_t SNRdB, uint16_t bitW, uint16_t n_samples, float resolution)
void MmwDemo_dataPathDeleteSemaphore(DSS_DataPathObj *obj)
Millimeter Wave Demo CFAR Configuration.
MmwDemo_1D_DopplerLines_t detDopplerLines
Detected Doppler lines.
void MmwDemo_dataPathConfigFFTs(DSS_DataPathObj *obj)
uint16_t currentIndex
starting index for the search for next active Doppler line
Parameters of CFAR detected object during the first round of CFAR detections.
cmplx16ImRe_t * azimuthModCoefs
Pointer to single point DFT coefficients used for Azimuth processing.
int16_t x
x - coordinate in meters. Q format provides the bitwidth.
int16_t sinAzim
wx sin(Azim). Q format provides the bitwidth.
int16_t * selectedIndxArr
uint16_t minRange
minimum range at which a target is detected ( in xyzOutputQFormat precision).
Parameters of CFAR detected object during the second round of CFAR detections.
uint32_t MmwDemo_pow2roundup(uint32_t x)
void MmwDemo_dataPathInit1Dstate(DSS_DataPathObj *obj)
clusteringDBscanOutput_t dbScanReport
The dBscan clustering result structures (holds pointers to the result).
error code for clusteringDBscan.
uint32_t activeFrameCPULoad
CPU Load during active frame period - i.e. chirping.
cmplx16ReIm_t * radarCube
Pointer to Radar Cube memory in L3 RAM.
float * trackerQvecList
Process noise constants.
cmplx32ReIm_t * antInp
input for Azimuth FFT
cycleLog_t cycleLog
DSP cycles for chirp and interframe processing and pending on EDMA data transferes.
void populateOutputs(DSS_DataPathObj *obj)
uint32_t chirpProcessingEndTime
Chirp processing end time.
float convertSNRLinToVar(uint16_t SNRLin, uint16_t bitW, uint16_t n_samples, float resolution)
MmwDemo_objRaw1D_t * detObj1DRaw
Detected objects after first pass in Doppler direction.
void parkingAssistInit(DSS_DataPathObj *obj)
uint32_t interFrameWaitTime
total wait time for 2D and 3D EDMA data transfer
struct clusteringDBscanReportForTx_t clusteringDBscanReportForTx
Structure for each cluster information report .
void MmwDemo_dataPathConfigAzimuthHeatMap(DSS_DataPathObj *obj)
cmplx32ReIm_t * azimuthIn
input for Azimuth FFT
uint16_t numVirtualAntAzim
number of virtual azimuth antennas
uint16_t * sumAbsRange
input buffer for CFAR processing from the detection matrix
uint16_t * parkingAssistBinsState
Filtered result of the nearest object as a function of azimuth.
void MmwDemo_processChirp(DSS_DataPathObj *obj, uint8_t subframeIdx)
struct KFtrackerInstance KFtrackerInstance_t
Struct for the tracker configuration, and pointers to scratch buffers.
uint16_t numDopplerBins
number of doppler bins
uint16_t numAngleBins
number of angle bins
uint32_t interChirpProcessingTime
total processing time during all chirps in a frame excluding EDMA waiting time
uint16_t rangeIdx
Range index.
uint32_t interFrameProcessingEndMargin
Inter frame processing end margin in number of cycles before due time to start processing first chirp...
clusteringDBscanErrorCodes
error code for clusteringDBscan.
uint8_t log2numVirtAnt
log2 of the number of virtual antennas.
uint16_t * cfarDetObjSNR
CFAR output objects' SNR buffer.
uint16_t dBScanNeighbourLim
void MmwDemo_dataPathConfigBuffers(DSS_DataPathObj *obj, uint32_t adcBufAddress)
uint16_t numChirpsPerChirpType
number of chirps per chirp type
int16_t y
y - coordinate in meters. Q format provides the bitwidth.
float maxUnambiguousVel
maximum unambiguous velocity (without algorithmic improvements) in meters/sec
float * azimuthMagSqr
output of Azimuth FFT magnitude squared
uint8_t chirpThreshold
Chirp Threshold configuration used for ADCBUF driver.
uint32_t chirpProcessingEndMarginMin
Chirp processing end margin in number of cycles before due time to start processing next chirp,...
float rangeResolution
range resolution in meters
uint16_t peakVal
Peak value.
struct MmwDemo_1D_DopplerLines MmwDemo_1D_DopplerLines_t
Active Doppler lines, lines (bins) on which the CFAR detector detected objects during the detections ...
uint32_t pruneTrackingInput(trackingInputReport_t *trackingInput, uint32_t numCluster)
float * trackerScratchPadFlt
Pointer to tracker scratch pad for floats.
Detected object estimated parameters to be transmitted out.
uint16_t parkingAssistMinRange
minimum range to look for obstacles. .
struct trackingReportForTx_t trackingReportForTx
Structure for tracking report.
#define N_UNIQ_ELEM_IN_SYM_COVMAT
Active Doppler lines, lines (bins) on which the CFAR detector detected objects during the detections ...
void MmwDemo_interFrameProcessing(DSS_DataPathObj *obj, uint8_t subframeIdx)
uint16_t rangeSNRdB
Range SNR (dB)
cmplx32ReIm_t * azimuthTwiddle32x32
twiddle factors table for Azimuth FFT
trackingReportForTx * trackerOpFinal
Final list of tracked objects for transmission.
uint16_t numTxAntennas
number of transmit antennas
uint16_t numAdcSamples
number of ADC samples
EDMA_transferControllerErrorInfo_t EDMA_transferControllerErrorInfo
EDMA transfer controller error information.
struct clusteringDBscanConfig clusteringDBscanConfig_t
Structure element of the list of descriptors for clusteringDBscan configuration.
uint16_t * parkingAssistBins
Nearest object as a function of azimuth.
int32_t * window2D
window coefficients for 2D FFT
uint16_t numChirpsPerFrame
number of chirps per frame
clusteringDBscanInstance_t dbScanInstance
The dBscan clustering configuration structure.
uint16_t * dbscanOutputDataIndexArray
Pointer to dBScan index array.
cmplx16ReIm_t * dstPingPong
ping pong buffer for 2D from radar Cube
uint16_t * cfarDetObjIndexBuf
CFAR output objects index buffer.
cmplx16ImRe_t azimuthModCoefsTwoThirdBin
Half bin needed for doppler correction as part of Azimuth processing.
uint16_t numActiveTrackers
number of active trackers.
int16_t * window1D
window coefficients for 1D FFT
struct MmwDemo_detectedObjForTx_t MmwDemo_detectedObjForTx
Detected object estimated parameters to be transmitted out.
uint16_t * log2Abs
log2 absolute computation output buffer
MmwDemo_detectedObjActual * detObj2D
Detected objects after second pass in Range direction. These objects are send out as point clouds.
Struct for the tracker configuration, and pointers to scratch buffers.
MmwDemo_CfarCfg cfarCfgRange
CFAR configuration in Range direction.
KFtrackerInstance_t trackerInstance
Tracking configuration structure.
uint16_t range
Range (in meters * (1 << xyzOutputQFormat))
uint16_t cfarCfgRange_minIndxToIgnoreHPF
The HPF can mess up the noise floor computation. So for a certain number of indices,...
uint16_t txAntennaCount
chirp counter modulo number of tx antennas
int16_t x
x - coordinate in meters. Q format provides the bitwidth.
cmplx32ReIm_t * elevationOut
output of Elevation FFT
uint16_t numDetObj
Number of detected objects.
uint8_t sinAzimQFormat
Q format of the sin of the azimuth.
clusteringDBscanReport_t * dbscanOutputDataReport
Pointer to dBScan output Report array.
Structure for tracking report.
uint32_t interFrameProcessingEndTime
Inter frame processing end time.
cmplx32ImRe_t * dcRangeSigMean
Pointer to DC range signature compensation buffer.
uint16_t * detMatrix
Pointer to range/Doppler log2 magnitude detection matrix in L3 RAM.
float quadraticInterpFltPeakLoc(float *restrict y, int32_t len, int32_t indx)
uint32_t interFrameProcessingTime
total processing time for 2D and 3D excluding EDMA waiting time
cmplx16ReIm_t * fftOut1D
1D FFT output
uint16_t numDetObjRaw
Number of detected objects.
float covmat[N_UNIQ_ELEM_IN_SYM_COVMAT]
clusteringDBscanReport_t * report
void MmwDemo_waitEndOfChirps(DSS_DataPathObj *obj, uint8_t subframeIdx)
cmplx32ReIm_t * azimuthOut
output of Azimuth FFT
float velResolution
velocity resolution in meters/sec
Structure for each cluster information report .
int16_t velDisambFacValidity
velocity disambiguation factor
cmplx16ReIm_t * adcDataIn
ADCBUF input samples in L2 scratch memory.
int16_t y
y - coordinate in meters. Q format provides the bitwidth.
uint16_t rangeSNRdB
SNR of the peak in the range dimension.
int16_t speed
Doppler (m/s in oneQformat)
MmwDemo_detectedObjForTx * detObjFinal
Final list of detected object for transmission.
int16_t z
z - coordinate in meters. Q format provides the bitwidth.
uint32_t transmitOutputCycles
time to transmit out detection information (in DSP cycles)
uint16_t numRangeBins
number of range bins
int32_t MmwDemo_dataPathConfigEdma(DSS_DataPathObj *obj)
struct cycleLog_t_ cycleLog_t
DSP cycle profiling structure to accumulate different processing times in chirp and frame processing ...
uint16_t peakVal
Peak value.
Detected object estimated parameters.
uint8_t xyzOutputQFormat
Q format of the output x/y/z coordinates.
#define DBSCAN_ERROR_CODE_OFFSET
float invOneSinAzimFormat
inverse of the oneQformat
uint32_t chirpProcessingEndMarginMax
Chirp processing end margin in number of cycles before due time to start processing next chirp,...
uint16_t dopplerSNRdB
SNR of the peak in the doppler dimension.
uint8_t * dBscanScratchPad
Pointer to dBScan scratch pad.
MmwDemo_CalibDcRangeSigCfg calibDcRangeSigCfg
DC Range antenna signature callibration configuration.
float invOneQFormat
inverse of the oneQformat
uint16_t dopplerIdx
Doppler index.
uint16_t * sumAbs
accumulated sum of log2 absolute over the antennae
Structure element of the list of descriptors for clusteringDBscan configuration.
Structure of clustering output.
int16_t speed
relative velocity (in meters/sec * (1 << xyzOutputQFormat))
uint8_t log2NumAvgChirps
log2 of number of averaged chirps
struct clusteringDBscanOutput clusteringDBscanOutput_t
Structure of clustering output.
int16_t * freeTrackerIndxArray
cmplx32ReIm_t * twiddle32x32_2D
twiddle table for 2D FFT
struct KFstate KFstate_t
Kalman filter state.
uint8_t processingPath
Processing path - either point-cloud or max-vel enhancement.
uint32_t interChirpWaitTime
total wait time for EDMA data transfer during all chirps in a frame
struct clusteringDBscanInstance clusteringDBscanInstance_t
error code for clusteringDBscan.
uint16_t threshold
SNR threshold (dB) for the range.
uint16_t chirpTypeCount
chirp counter modulo number of subframe
struct maxVelEnhStruct_t_ maxVelEnhStruct_t
pre-computed parameters of the max-velocity-enhancement.
struct MmwDemo_objRaw1D MmwDemo_objRaw1D_t
Parameters of CFAR detected object during the first round of CFAR detections.
uint16_t dopplerSNRdB
Doppler SNR (dB)
Millimeter Wave Demo Data Path Information.
MmwDemo_CfarCfg cfarCfgDoppler
CFAR configuration in Doppler direction.
float invVelResolutionSlowChirp
pre-computed parameters of the max-velocity-enhancement.
maxVelEnhStruct_t maxVelEnhStruct
Max-velocity constants.
struct clusteringDBscanReport clusteringDBscanReport_t
Structure for each cluster information report .
clusteringDBscanReportForTx * clusterOpFinal
Final list of clusters for transmission.
float invNumAngleBins
inverse of the numAngleBins
cmplx32ReIm_t * elevationIn
input for Elevation FFT
struct DSS_DataPathObj_t DSS_DataPathObj
Millimeter Wave Demo Data Path Information.
uint8_t padding
padding. .
float velResolutionFastChirp
uint16_t dopplerIdx
Doppler index.
void MmwDemo_addDopplerCompensation(int32_t dopplerIdx, int32_t numDopplerBins, uint32_t *azimuthModCoefs, uint32_t *azimuthModCoefsThirdBin, uint32_t *azimuthModCoefsTwoThirdBin, int64_t *azimuthIn, uint32_t numAnt, uint32_t numTxAnt, uint16_t txAntIdx)
Function Name : MmwDemo_DopplerCompensation.
RangeDependantThresh_t peakValThresholds[MAX_NUM_RANGE_DEPENDANT_SNR_THRESHOLDS]
SNR thresholds as a function of range.
uint16_t sinAzimSNRLin
omega SNR (linear scale)
cmplx16ImRe_t azimuthModCoefsThirdBin
Half bin needed for doppler correction as part of Azimuth processing.
trackingInputReport_t * trackingInput
Pointer to dBScan output used as input to the tracker.
uint16_t range
Range (meters in oneQformat)
int16_t speed
Doppler index.
uint16_t rangeIdx
Range index.
DSP cycle profiling structure to accumulate different processing times in chirp and frame processing ...
MmwDemo_MultiObjBeamFormingCfg multiObjBeamFormingCfg
Multi object beam forming configuration.
struct MmwDemo_objRaw2D MmwDemo_objRaw2D_t
Parameters of CFAR detected object during the second round of CFAR detections.
void MmwDemo_XYcalc(DSS_DataPathObj *obj, uint32_t objIndex, uint16_t azimIdx, float *azimuthMagSqr)
uint32_t interFrameProcCycles
number of processor cycles between frames excluding processing time to transmit output on UART
cmplx16ReIm_t * ADCdataBuf
pointer to ADC buffer
cmplx16ImRe_t azimuthModCoefsHalfBin
Half bin needed for doppler correction as part of Azimuth processing.
uint16_t minPointsInCluster
uint32_t * dopplerLineMask
Doppler line bit mask of active (CFAR detected) Doppler bins in the first round of CFAR detections in...
uint16_t numVirtualAntElev
number of virtual elevation antennas
uint8_t log2NumDopplerBins
log 2 of number of doppler bins
int16_t z
z - coordinate in meters. Q format provides the bitwidth.
EDMA_errorInfo_t EDMA_errorInfo
EDMA error Information when there are errors like missing events.
Constants for the Extended Kalman Filter.
uint16_t * sumAbsSlowChirp
accumulated sum of log2 absolute over the antennae
Millimeter Wave Demo DC range signature compensation.
struct trackingInputReport trackingInputReport_t
Input to tracking from the clustering output.
uint16_t maxNumObj2DRaw
number of objects to be detected in 2D-CFAR.
struct SNRThresholds RangeDependantThresh_t
These parameters allow the SNR requirements to be varied as a function of range.
uint16_t numVirtualAntennas
number of virtual antennas
int32_t MmwDemo_dataPathInitEdma(DSS_DataPathObj *obj)
void MmwDemo_XYZcalc(DSS_DataPathObj *obj, uint32_t objIndex, uint16_t azimIdx, float *azimuthMagSqr)
uint16_t chirpCount
chirp counter modulo number of chirps per frame
uint8_t subframeIndx
index of the subframe to which this object belongs
uint8_t dcRangeSigCalibCntr
DC range signature calibration counter.
clusteringDBscanReport_t * dbScanState
Pointer to dBScan output Report array.
uint8_t parkingAssistNumBinsLog2
log2 of the number of bins for the parkingAssist module (used for scaling operations).
uint8_t parkingAssistNumBins
Number of bins for the parkingAssist module.
MmwDemo_timingInfo_t timingInfo
Timing information.
cmplx16ReIm_t * twiddle16x16_1D
twiddle table for 1D FFT
struct MmwDemo_timingInfo MmwDemo_timingInfo_t
Timing information.
uint16_t dopplerBinCount
chirp counter modulo number of Doppler bins
int16_t * trackerScratchPadShort
Pointer to tracker scratch pad for int16_t.
ADCBuf_Handle adcbufHandle
ADCBUF handle.
uint16_t * parkingAssistBinsStateCnt
The 'age' of the filtered result of the parking state. Added to make the 'smoother' visually.
struct MmwDemo_detectedObjActual_t MmwDemo_detectedObjActual
Detected object estimated parameters.
uint16_t parkingAssistMaxRange
maximum range to look for obstacles. .
uint16_t rangeIdx
Range index.
uint16_t numRxAntennas
Number of receive channels.
uint16_t dopplerIdx
Doppler index.